In the modern world of mobile app development, maintaining strong security is crucial. With the
growing complexity of applications and their integration with various services, protecting user data
and maintaining application integrity is essential for developers and businesses alike. React Native, a
widely-used framework for building cross-platform mobile apps, presents both opportunities and
challenges when it comes to security. This blog aims to provide practical advice on how to enhance
security in React Native applications, focusing on strategies to protect your app from potential
threats. Whether you’re involved in React Native app development or managing a team of
developers, understanding and implementing effective security measures is key to safeguarding your
application and its data.
What is React Native?
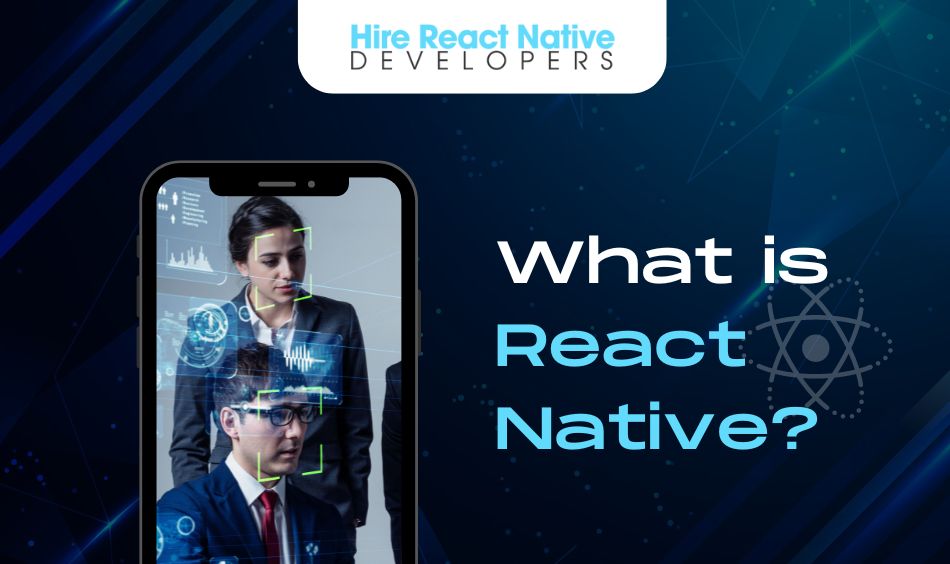
React Native is an open-source framework created by Facebook for developing mobile applications
using JavaScript and React. It allows developers to write code once and deploy it on both iOS and
Android platforms. This approach leverages native components to deliver a high-quality user
experience.
Key Features of React Native
Cross-Platform Compatibility: React Native enables the creation of apps that work on both iOS and
Android from a single codebase. This reduces the need for separate development teams and
minimizes duplicated effort.
Native Performance: Unlike some hybrid frameworks that rely on web views, React Native uses actual
native components. This helps in achieving a performance level that is closer to native apps.
Hot Reloading: React Native supports hot reloading, which allows developers to see the results of
code changes instantly without recompiling the entire application. This feature speeds up the
development process and makes debugging more efficient.
Rich Ecosystem: The framework benefits from a wide range of libraries and tools, which can extend
its functionality and improve development efficiency.
Also read : What is React Bootstrap? Learn the Basics
What Makes React Native Unique?
React Native stands out in mobile development due to its distinct approach to building cross-platform
apps. Here are some aspects that set it apart:
JavaScript and React Integration : React Native combines JavaScript, a widely used programming
language, with React, a powerful library for building user interfaces. This integration allows
developers to apply familiar concepts and tools in mobile app development.
Native Components : Instead of using web views to render content, React Native employs native
components. This approach leads to apps that are more responsive and provide a smoother user
experience, closely resembling the performance of native apps.
Unified Codebase : One of the main advantages of React Native is the ability to maintain a single
codebase for both iOS and Android platforms. This not only accelerates development but also
simplifies maintenance and updates.
Community and Ecosystem : The framework benefits from a strong and active community that
continuously contributes to its development. This results in regular updates and a rich array of thirdparty libraries and tools.
Are React Native Apps Secure?
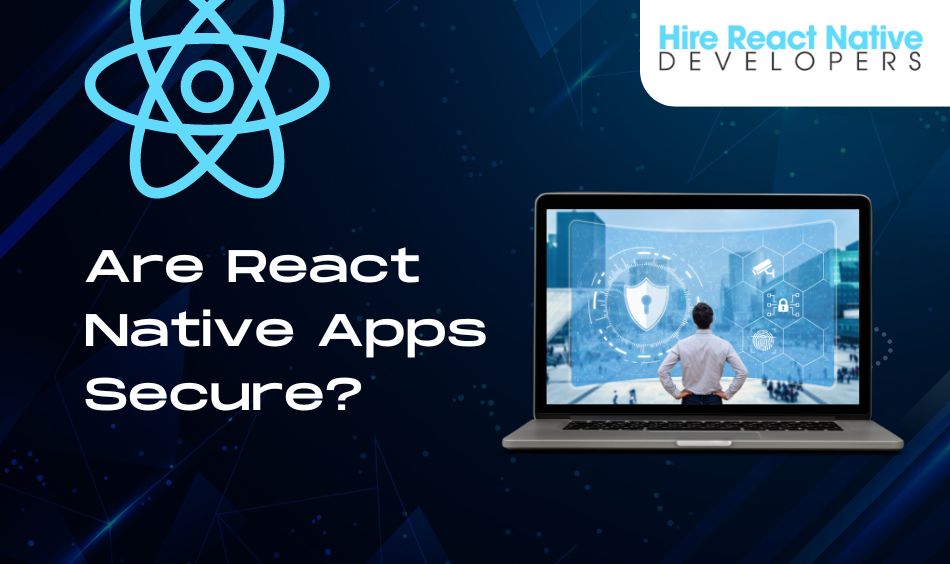
The security of React Native apps depends significantly on the practices of React Native app
developers and the measures they implement. While React Native provides some built-in security
features, such as encryption and secure storage, it is essential for developers to actively address
potential vulnerabilities and follow best practices. Common risks include data leakage and insecure
storage, which require careful management. By adhering to security guidelines and keeping up with
the latest threats, React Native app developers can better safeguard their applications and protect
user data.
Best Security Practices to Keep in Mind While Developing an App with React Native
Implementing effective security measures in React Native apps is crucial for protecting user data and
maintaining the integrity of your application. Below are key practices to follow during development to
safeguard your app from security vulnerabilities.
Android-Specific Security Practices
1 Use ProGuard and R8
ProGuard and R8 are tools provided by Android for code shrinking and obfuscation. They make your
code harder to reverse-engineer by removing unused code and renaming classes and methods.
Enabling these tools in your build.gradle file helps in protecting your source code from unauthorized
analysis. Make sure to test your app thoroughly after applying obfuscation, as it can sometimes
introduce issues if not configured correctly.
2 Secure Shared Preferences
Shared Preferences is commonly used to store simple data in Android. However, it is not suitable for
sensitive information. Instead, use Android’s EncryptedSharedPreferences, which encrypts the data
before saving it, making it inaccessible to unauthorized users or apps.
3 Implement Android SafetyNet
SafetyNet is a set of APIs that help you evaluate the security and compatibility of the user’s device. It
can detect if the device is rooted or compromised, which could potentially undermine the security of
your app. By integrating SafetyNet, you can ensure that your app operates within a secure
environment.
4 Use Android Keystore System
The Android Keystore system provides a way to securely generate and store cryptographic keys. By
storing keys in the Keystore, you prevent unauthorized access to these keys and protect sensitive data
encryption. This system helps manage cryptographic operations without exposing keys directly to
your app code.
iOS-Specific Security Practices
1 Secure Keychain Access
The iOS Keychain is designed for securely storing sensitive information such as passwords, tokens, and
encryption keys. Unlike other storage mechanisms, the Keychain encrypts data and provides access
control to protect it from unauthorized access. Use Keychain services for all sensitive data storage
needs.
2 Enable App Transport Security (ATS)
App Transport Security enforces the use of HTTPS for network communications. By requiring secure
connections, ATS helps protect data during transmission and prevents man-in-the-middle attacks.
Ensure that ATS is enabled in your Info.plist file and properly configured to enforce secure
connections.
3 Implement Code Obfuscation
While iOS is less susceptible to reverse engineering, obfuscating your code can add an extra layer of
protection. Tools like SwiftShield can be used to obscure your Swift and Objective-C code, making it
more difficult for attackers to analyze and exploit.
4 Check for Jailbroken Devices
Jailbroken devices can bypass iOS security measures, making them vulnerable to attacks. Implement
code checks to detect if the device is jailbroken and handle such situations appropriately, such as
restricting access or providing warnings to users.
Enable Hermes
Hermes is a lightweight JavaScript engine developed by Facebook specifically for React Native. It is
designed to improve performance by reducing memory usage and speeding up execution. While
Hermes does not directly address security issues, its efficient operation contributes to a more stable
app environment. To enable Hermes, update your React Native configuration and ensure you keep it
updated to benefit from the latest performance and security improvements.
SSL Pinning with React Native
SSL pinning is a security measure that helps protect against man-in-the-middle (MitM) attacks by
validating that the server’s SSL certificate matches a known and trusted certificate. This process
involves embedding the certificate or its public key within the app. Use libraries such as react-nativessl-pinning to implement SSL pinning, ensuring that your app only communicates with trusted servers
and encrypting the data transmitted between your app and the server.
Avoid Using Deprecated Libraries
Using outdated or deprecated libraries can introduce vulnerabilities into your application. Deprecated
libraries may no longer receive updates or security patches, leaving your app exposed to known
vulnerabilities. Regularly review your project dependencies and update them to their latest versions
to benefit from security improvements and bug fixes.
Storage Security
1 Secure Local Storage
Avoid storing sensitive data in plain text or in locations accessible by other apps or users. Use React
Native’s secure storage options, such as @react-native-community/async-storage with encryption for
added security. Ensure that all data stored locally is properly encrypted to prevent unauthorized
access.
2 Data Encryption
Encrypt sensitive data before storing it on the device. Implement encryption algorithms to protect
data, ensuring that even if the device is compromised, the encrypted data remains secure. Use
libraries like react-native-encrypted-storage for secure encryption and storage of sensitive
information.
3 Use Secure Storage Libraries
Consider using libraries specifically designed for secure storage, such as react-native-sensitive-info.
These libraries provide secure storage mechanisms for sensitive data and help ensure that your app
complies with best practices for data protection.
XSS Attacks
Cross-Site Scripting (XSS) attacks involve injecting malicious scripts into web applications. While React
Native apps are less prone to XSS compared to web apps, it is important to sanitize any usergenerated content before rendering it. Use libraries that automatically handle content sanitization
and validate all inputs to prevent XSS attacks.
Deep Linking
Deep linking allows users to navigate directly to specific content within your app. To prevent security
issues, validate and sanitize all deep link URLs to ensure they do not lead to unauthorized or restricted
areas of the app. Implement proper checks and authentication to ensure that users cannot exploit
deep linking to access sensitive content.
Secure API Endpoints
1 Use HTTPS for API Calls
All communication between your app and backend services should occur over HTTPS to protect data
in transit. HTTPS encrypts the data transmitted between the app and server, reducing the risk of
interception and tampering.
2 Authenticate API Requests
Implement strong authentication mechanisms for API requests to verify the identity of users and
applications. Use methods such as OAuth tokens, JWT (JSON Web Tokens), or API keys to ensure that
only authorized users can access your API endpoints.
3 Rate Limiting
Implement rate limiting to control the number of requests that can be made from a single IP address
or user within a given timeframe. Rate limiting helps prevent abuse and reduces the risk of denial-ofservice (DoS) attacks, protecting your backend services from being overwhelmed.
Authenticate SHA256
SHA256 is a cryptographic hash function used to ensure data integrity. Implement SHA256 hashing to
verify that data has not been altered during transmission or storage. Use SHA256 in combination with
other security measures, such as encryption, to provide a more secure environment for your app.
Keep a Check for Jailbroken and Rooted Phones
1 Detect Rooted/Jailbroken Devices
Implement checks within your app to detect if the device is rooted (Android) or jailbroken (iOS). Such
devices often have compromised security and can bypass standard app protections.
2 Handle Compromised Devices
Decide on the actions your app should take if it detects a rooted or jailbroken device. Options include
restricting access, providing warnings, or preventing app usage altogether. This helps protect your
app’s security by ensuring it only operates in a secure environment.
Also read : Step-by-Step Guide to React Native IoT App Development
Hire React Native App Developers
Choosing the right development team is crucial to the success of your React Native application. When
hiring React Native app developers, it’s important to consider their expertise, experience, and
understanding of security practices. Skilled developers not only build functional and engaging
applications but also implement essential security measures to protect your app from potential
threats.
Assess Expertise in React Native
Ensure that the developers you hire have extensive experience with React Native. Their familiarity
with the framework will help them leverage its full potential while maintaining best practices for
security. Experienced developers can efficiently address issues and incorporate advanced features
into your app.
Evaluate Their Approach to Security
Security should be a priority for any developer working on your app. Look for developers who are
knowledgeable about React Native security practices and who follow industry standards to protect
user data. They should be well-versed in secure coding practices, data encryption, and safe API usage.
Review Their Previous Projects
Examine the developers’ previous work to assess their capability in handling complex projects.
Reviewing case studies or sample apps can provide insights into their ability to build secure and highquality applications. This will also give you an idea of their problem-solving skills and creativity.
Consider Their Communication Skills
Effective communication is key to a successful development process. Hire developers who are not
only technically proficient but also able to clearly articulate their ideas and solutions. Good
communication ensures that your requirements and security needs are understood and implemented
correctly.
Choose a Reliable Development Partner
A reliable development partner can make a significant difference in the outcome of your project. Shiv
Technolabs is one such company that specializes in React Native app development. Their team of
experienced developers brings both technical expertise and a strong commitment to security
practices. By partnering with Shiv Technolabs, you can rest assured that your app will be developed
with the highest standards of quality and security in mind.
Hiring the right React Native app developers, such as those from Shiv Technolabs, is a critical step in
building a successful and secure application. By focusing on developers’ expertise, security awareness,
and communication skills, you can create an app that not only meets user expectations but also
stands strong against potential security threats.